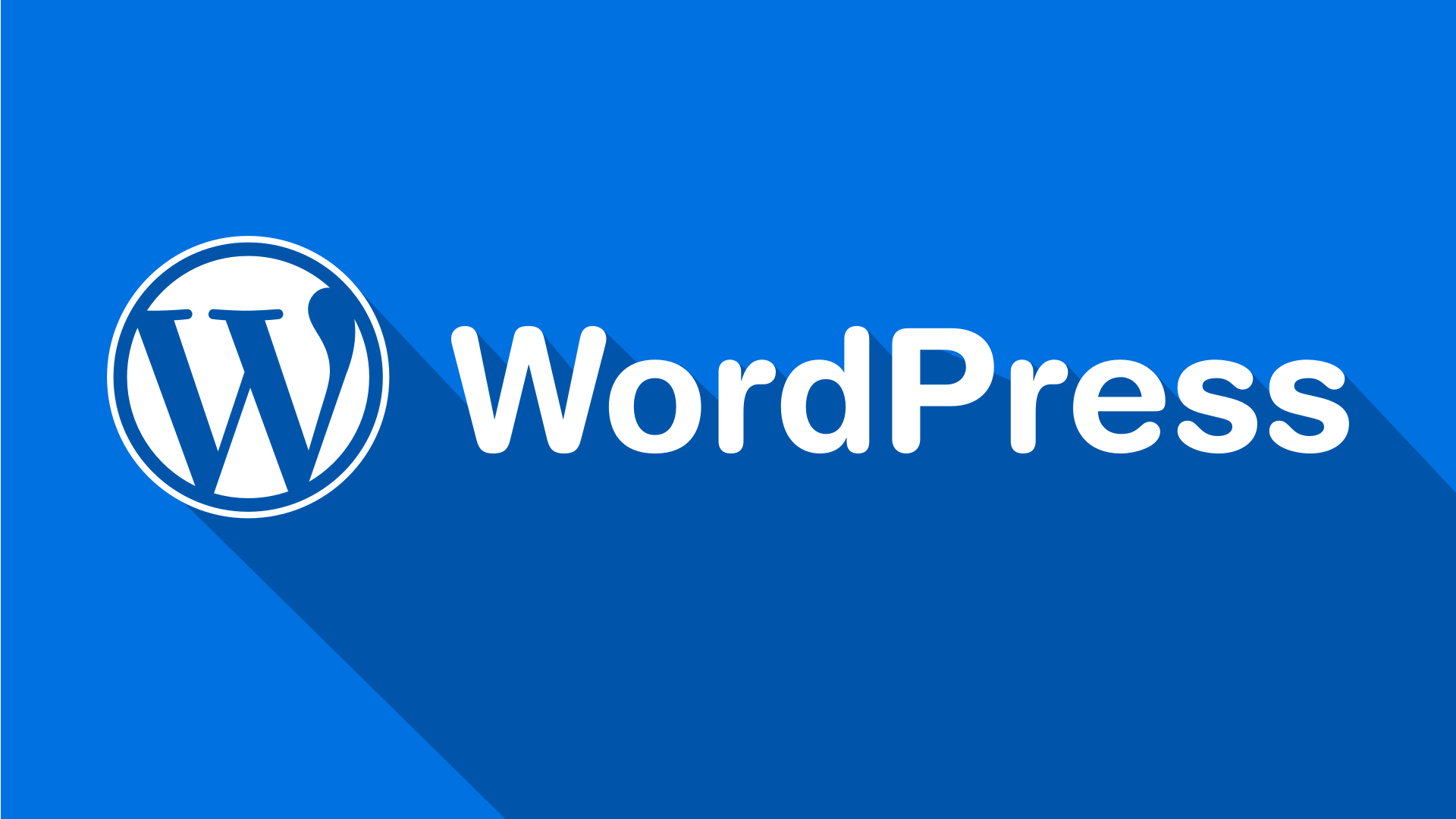
While designing and trying to create the gallery section of the website for my gaming screenshots and other images I ran into a tiny problem with how WordPress handles files uploads. The problem was that when you upload a file to the database with the media library it sets the “post_date” and “post_modified” fields in the database to the date you uploaded the file. This creates a problem when trying to filter screenshots from anywhere between three years to over a decade ago based on the date in the database, and scanning the files directly for the ones that had the correct creation and last modified dates is an expensive and inefficient operation in and of itself, it’s the reason we have this information stored in a database.
To solve this I made a small little one file plugin that adds a button to the WordPress media library tab that will scan the media files in the database, and update their post date and modified date to the last time the actual file was modified. Now that this problem is solved I will be able to work on the gallery sections of the websites for my gaming screenshots and other things. Those should hopefully be live in a few days if all goes to plan, I will make another post announcing when they are live. Below is the code I used to fix the problem I had, it is far from optimal but it serves its purpose for now, please feel free to use it for your own projects and websites if it helps with a similar situation or problem. (https://gist.github.com/XerShade/37cb73ecad6b294571739c2c513424e2)
<?php
/*
Plugin Name: Sync Media Dates
Description: Adds a button under the Media Library tab to sync the post date and post date GMT of media files to be the date the file was last modified.
Version: 1.0
Author: XerShade
*/
function sync_media_dates_page() {
add_media_page(
'Sync Media Dates',
'Sync Media Dates',
'manage_options',
'sync-media-dates',
'sync_media_dates_callback'
);
}
add_action('admin_menu', 'sync_media_dates_page');
function sync_media_dates_callback() {
if (isset($_POST['sync_media_dates_nonce']) && wp_verify_nonce($_POST['sync_media_dates_nonce'], 'sync_media_dates_action')) {
// Run the code to sync media dates.
sync_media_dates();
echo '<div class="updated"><p>Media dates synced successfully!</p></div>';
}
?>
<div class="wrap">
<h1>Sync Media Dates</h1>
<form method="post">
<?php wp_nonce_field('sync_media_dates_action', 'sync_media_dates_nonce'); ?>
<p>This will sync the post date and post date GMT of all media files to be the date the file was last modified.</p>
<p><button type="submit" class="button button-primary">Sync Media Dates</button></p>
</form>
</div>
<?php
}
function sync_media_dates() {
$media_query = new WP_Query(
array(
'post_type' => 'attachment',
'posts_per_page' => -1,
'post_status' => 'any',
)
);
if ($media_query->have_posts()) {
while ($media_query->have_posts()) {
$media_query->the_post();
$attachment_id = get_the_ID();
$file_path = get_attached_file($attachment_id);
$file_created = filectime($file_path);
$file_modified = filemtime($file_path);
// Sync both post_date and post_date_gmt to the file creation date.
wp_update_post(
array(
'ID' => $attachment_id,
'post_date' => date('Y-m-d H:i:s', $file_modified),
'post_date_gmt' => get_gmt_from_date(date('Y-m-d H:i:s', $file_modified)),
'post_modified' => date('Y-m-d H:i:s', $file_modified),
'post_modified_gmt' => get_gmt_from_date(date('Y-m-d H:i:s', $file_modified)),
)
);
}
wp_reset_postdata();
}
}
I am providing this code as is and I am not offering any support to expanding it or changing its functionality. It was created to serve a simple purpose for a very specific problem I had for my website. If you would like to contribute to it then please post your changes on a GitHub gist and link it to me and I will review and update the code on my end. The latest code can be found at the following link in case I forget to update this post: https://gist.github.com/XerShade/37cb73ecad6b294571739c2c513424e2
Comments